Views 1,084
How to Create a Horizontal Scroll Effect with React and GSAP ScrollTrigger: Service Section Component
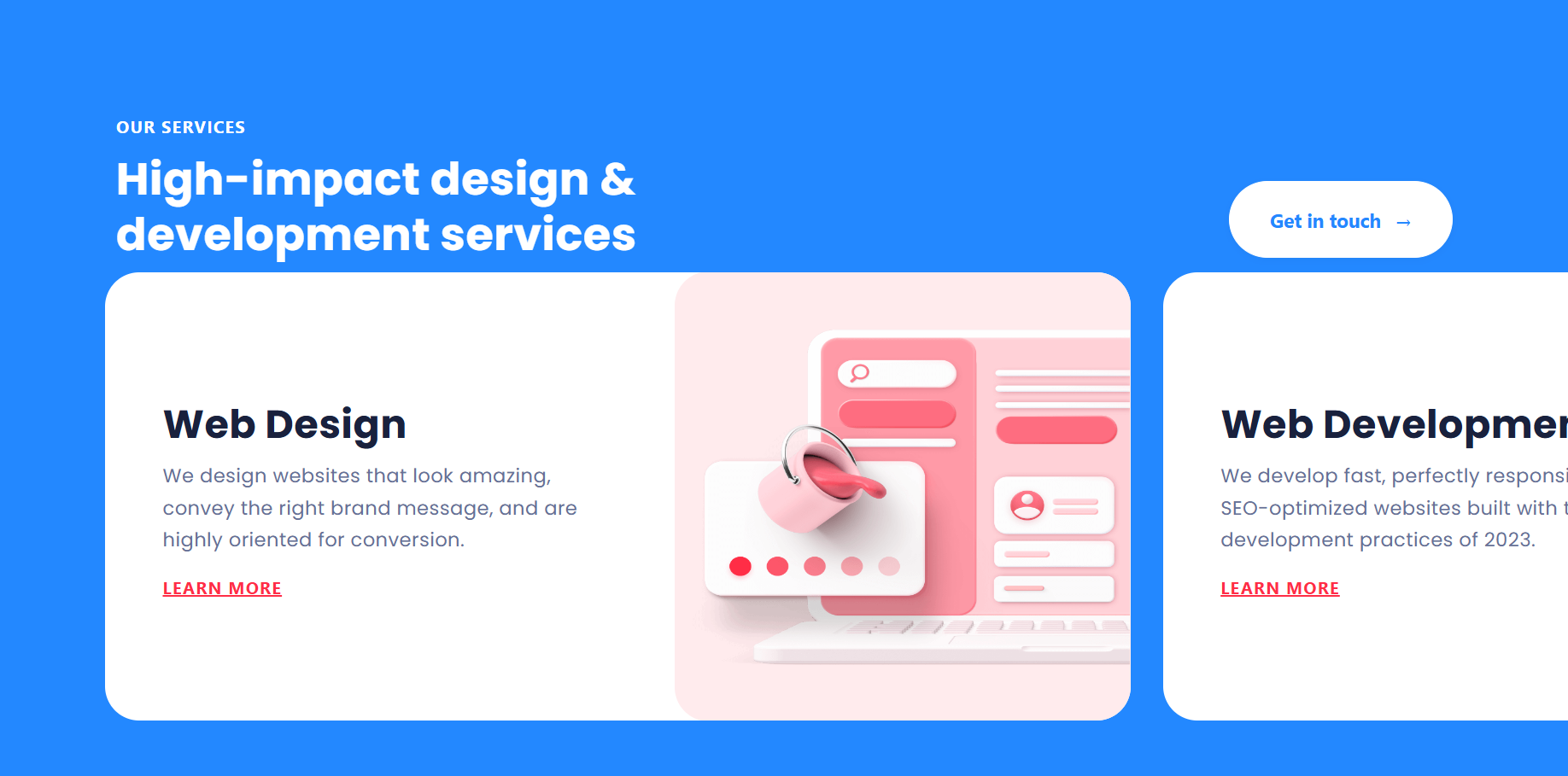
Horizontal Scroll Effect with React and GSAP ScrollTrigger: High interactive scroll trigger section for Services Creating interactive and engaging websites often requires adding unique scrolling effects. One popular effect is horizontal scrolling, which can be particularly effective for showcasing sections like services. In this article, we’ll walk you through how to create a horizontal scroll effect for a services section using React and GSAP ScrollTrigger.
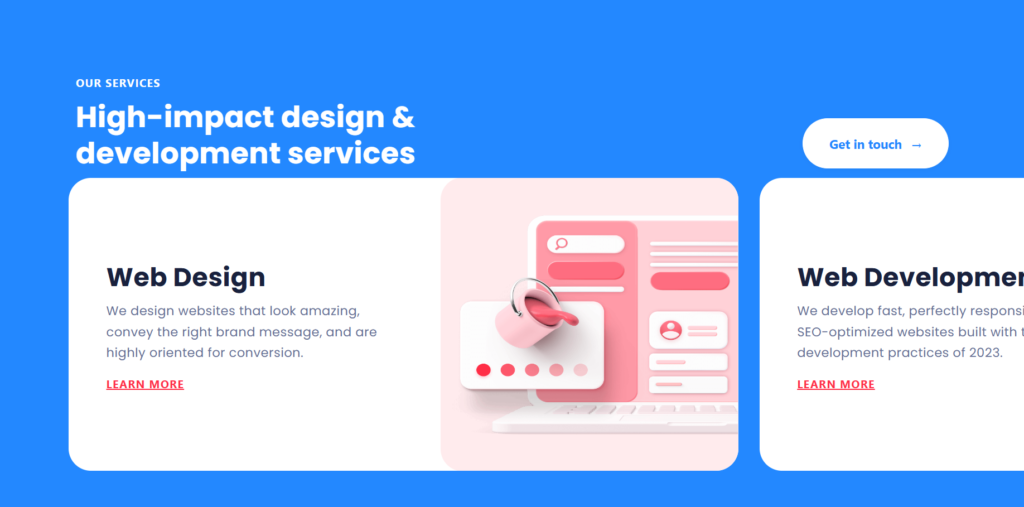
Note : Only for lap or desktop view; not recommended for mobile view.
Before going to build see live here – Horizontal scroll.
Why use horizontal Scrolling?
Horizontal scrolling can make your website more dynamic and interactive. It’s especially useful for:
- Showcasing Services: Display services in a unique, engaging manner.
- Portfolio Sections: Highlight different projects in a visually appealing way.
- Image Galleries: Create interactive photo galleries.
What are GSAP and ScrollTrigger?
GSAP (GreenSock Animation Platform) is a powerful JavaScript library for creating high-performance animations.
ScrollTrigger is a GSAP plugin that lets you create scroll-driven animations with ease.
Setting Up Your Project
First, ensure you have Node.js installed. Then, create a new React project:
Create react app: npm install -g create-react-app
Install latest GSAAP frameworks - npm install @gsap/react - read here
start with these imports
import React, { useRef } from "react";
import gsap from "gsap";
import ScrollTrigger from 'gsap/ScrollTrigger';
import { useGSAP } from "@gsap/react";
Creating the Horizontal Scroll Effect
- Set Up the Component Structure:Create a new component for your services section:jsxCopy code
.
function Horizontalscroll() {
const containerRef = useRef(); // Reference to the container
useGSAP(() => {
// Ensure GSAP plugins are registered
gsap.registerPlugin(ScrollTrigger);
// Target elements with the class "cards"
const cards = gsap.utils.toArray(".cards");
// Create the horizontal scrolling animation
gsap.to(cards, {
xPercent: -100 * (cards.length - 1),
ease: "none",
scrollTrigger: {
trigger: containerRef.current, // Use the ref as the trigger
pin: true,
anticipatePin: true,
stagger:{amount:-1},
scrub: true,
snap: 1 / (cards.length - 1),
end: () => "+=" + containerRef.current.offsetWidth, // Proper use of template literals
},
});
}, []); // Dependencies array if any dependencies needed, otherwise empty for setup only
return (
<section ref={containerRef} className="scroll-container">
<div>
<HeadingContent />
</div>
<div className="cards-container">
<your-item />
<your-item2 />
<your-item3 />
<your-item4 />
<your-item5 />
<your-item6 />
</div>
</section>
);
}
replace card items
- Add CSS for Styling:Create a CSS file for styling the services section:cssCopy cod
e.
.scroll-container{
background: #2388FF;
width: 100%;
height: 100vh;
padding: 110px 0px;
overflow: hidden;
}
.cards-contaiener{
grid-column-gap: 28px;
flex-direction: row;
display: flex;
padding-left: 100px;
}
- Integrate the Services Component: Use the
followin
g component in your main application
function App() {
return (
<>
<Hero />
<Parallax />
</>
);
}
How It Works
- Component Structure: We created a
Services
component with a container and a series of service items. - GSAP and ScrollTrigger Setup: In the
useEffect
hook, we initialize GSAP and ScrollTrigger to animate theservices
element horizontally as the user scrolls. - Pinning and Scrubbing: The
ScrollTrigger
plugin pins and scrubs the animation, making it smooth and interactive.
Final Thoughts
By following these steps, you can create a highly interactive horizontal scroll effect for your services section using React and GSAP ScrollTrigger. This effect can make your website more dynamic and engaging, providing a better user experience. Feel free to customize the styles and contents to fit your project’s needs.
Happy coding!
Github repo link – Horizontal scroll