Views 130
Image Reveal effect on hover on list-with gsap in Wordpress
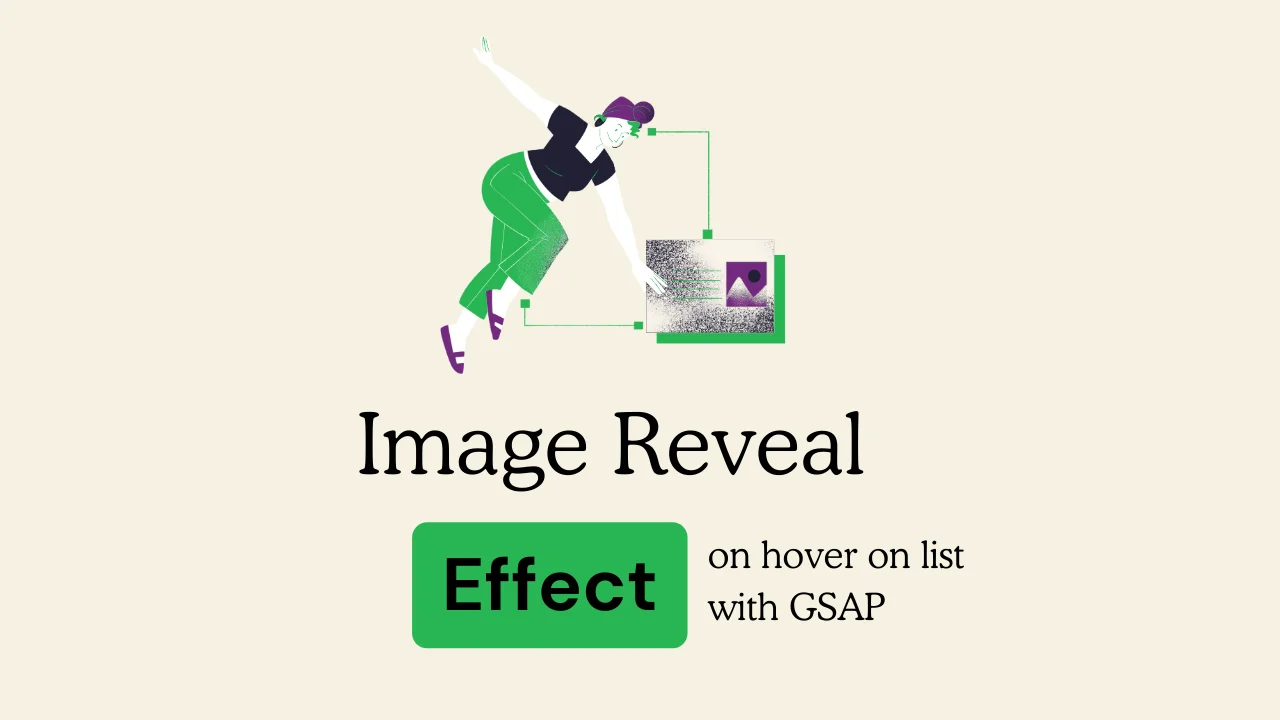
index
0%
Image Reveal effect-on hover on list-with gsap in Wordpress – Creating an engaging navigation menu enhances user experience, and GSAP (GreenSock Animation Platform) allows for smooth and professional animations. In this article, we will create a menu where an image appears and follows the cursor when hovering over menu items.
Image Reveal effect on hover on list-with gsap in wordpress
Read more about GSAP Expanding menu with html css js Clean animation for websites
Steps to Create a GSAP Menu Hover Image Reveal Effect
1. Set Up the HTML Structure
We need a basic menu with list items and an image container that will follow the cursor.
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Menu Hover Image Open Effect with Gsap in HTML CSS JS</title>
<link href="https://fonts.googleapis.com/css2?family=DM+Sans:wght@400;500;700&display=swap" rel="stylesheet">
<script src="https://unpkg.com/gsap@3/dist/gsap.min.js"></script>
<link rel="stylesheet" href="./style.css">
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.12.2/gsap.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.12.2/ScrollTrigger.min.js"></script>
</head>
<body>
<div id="fancyServices">
<div class="image__cursor"></div>
<div class="service__list">
<div class="service__item service__item-1">
<a class="service__item--inner" href="#">
<div class="service__title">
<h2>Branding</h2>
</div>
<div class="service__icon">
<i>-></i>
</div>
<div class="service__overlay"></div>
</a>
<div class="service__image">
<img src="https://thefallen.in/wp-content/uploads/2024/08/63407fbdc2d4ac5270385fd4_home-hero-image-paperfolio-webflow-template.svg" alt="">
</div>
<div class="hr__line"></div>
</div>
<div class="service__item service__item-2">
<a class="service__item--inner" href="#">
<div class="service__title">
<h2>Web Design</h2>
</div>
<div class="service__icon">
<i>-></i>
</div>
<div class="service__overlay"></div>
</a>
<div class="service__image">
<img src="https://assets-global.website-files.com/63360c0c2b86f80ba8b5421a/6340863899262a16cc4589d7_web-design-image-paperfolio-webflow-template.svg" alt="">
</div>
<div class="hr__line"></div>
</div>
<div class="service__item service__item-3">
<a class="service__item--inner" href="#">
<div class="service__title">
<h2>SEO & Social</h2>
</div>
<div class="service__icon">
<i>-></i>
</div>
<div class="service__overlay"></div>
</a>
<div class="service__image">
<img src="https://assets-global.website-files.com/63360c0c2b86f80ba8b5421a/63408706e5403a54a108b2d3_ui-ux-design-image-paperfolio-webflow-template.svg" alt="">
</div>
<div class="hr__line"></div>
</div>
<div class="service__item service__item-4">
<a class="service__item--inner" href="#">
<div class="service__title">
<h2>Marketing</h2>
</div>
<div class="service__icon">
<i>-></i>
</div>
<div class="service__overlay"></div>
</a>
<div class="service__image">
<img src="https://thefallen.in/wp-content/uploads/2024/08/63407fbdc2d4ac5270385fd4_home-hero-image-paperfolio-webflow-template.svg" alt="">
</div>
<div class="hr__line"></div>
</div>
<div class="service__item service__item-5">
<a class="service__item--inner" href="#">
<div class="service__title">
<h2>Copywriting</h2>
</div>
<div class="service__icon">
<i>-></i>
</div>
<div class="service__overlay"></div>
</a>
<div class="service__image">
<img src="https://thefallen.in/wp-content/uploads/2024/08/63407fbdc2d4ac5270385fd4_home-hero-image-paperfolio-webflow-template.svg" alt="">
</div>
<div class="hr__line"></div>
</div>
</div>
</div>
</body>
2. Style the Menu and Image Reveal with CSS & scss
We position the image-reveal div absolutely so it follows the cursor.
$colorBlack: #000;
body {
overflow: hidden;
background: #000;
color: white;
}
.image__cursor {
position: absolute;
width: 400px;
height: 600px;
top: 50%;
left: 50%;
margin: -100px 0 0 -100px;
background: no-repeat 50% 50%;
background-size: cover;
z-index: 1;
will-change: left, top, transform;
transform: scale(0.1);
transition: transform 0.3s ease, opacity 0.3s ease;
pointer-events: none;
}
#fancyServices {
padding: 60px 40px;
}
.service {
&__list {
overflow: hidden;
z-index: 2;
position: relative;
}
&__overlay {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
&__image {
opacity: 0;
visibility: hidden;
display: none;
}
&__item {
&--inner {
display: flex;
justify-content: space-between;
align-items: center;
text-decoration: none;
color: $colorBlack;
position: relative;
}
}
}
.hr__line {
height: 1px;
width: 100%;
background-color: #ddd;
}
3. Add GSAP Animations with JavaScript
We use GSAP to animate the image reveal effect on hover.
const cursor = document.querySelector('.image__cursor');
const overlayItems = document.querySelectorAll('.service__overlay');
const fancyServices = document.querySelector('#fancyServices');
// Move cursor with mouse or touch events relative to #fancyServices
function moveCursor(e) {
const rect = fancyServices.getBoundingClientRect(); // Get #fancyServices dimensions and position
const offsetX = e.pageX - rect.left; // Calculate relative X position
const offsetY = e.pageY - rect.top; // Calculate relative Y position
gsap.to(cursor, {
duration: 0.5,
left: offsetX,
top: offsetY,
delay: 0.03
});
}
// Update cursor background dynamically from each service item's image
document.querySelectorAll('.service__item').forEach((item) => {
item.addEventListener("mouseover", () => {
const imageSrc = item.querySelector('.service__image img').getAttribute('src');
cursor.style.backgroundImage = `url(${imageSrc})`;
});
});
let isCursorActive = false;
// Show cursor on overlay and enable movement
overlayItems.forEach((overlay) => {
overlay.addEventListener('mousemove', (e) => {
if (!isCursorActive) {
isCursorActive = true;
gsap.to(cursor, {
scale: 1,
autoAlpha: 1,
duration: 0.3
});
}
moveCursor(e);
});
overlay.addEventListener('touchmove', (e) => {
if (!isCursorActive) {
isCursorActive = true;
gsap.to(cursor, {
scale: 1,
autoAlpha: 1,
duration: 0.3
});
}
moveCursor(e.touches[0]);
});
// Hide cursor when leaving the overlay
overlay.addEventListener('mouseout', () => {
isCursorActive = false;
gsap.to(cursor, {
scale: 0.1,
autoAlpha: 0,
duration: 0.3
});
});
overlay.addEventListener('touchend', () => {
isCursorActive = false;
gsap.to(cursor, {
scale: 0.1,
autoAlpha: 0,
duration: 0.3
});
});
});
Conclusion
With GSAP, you can create an elegant menu hover effect where images dynamically appear and follow the cursor. This enhances interactivity and provides a modern, visually appealing user experience. Try customizing it further by adjusting animations, sizes, or adding transitions!
Happy coding! 🚀
Image Reveal effect on hover on list-with gsap in Wordpress
About Me
I’m Ganesh, a passionate web designer with over two years of experience. I love creating modern and user-friendly components for web builders like Framer, Elements, and WordPress. If you find this free header helpful, I’d appreciate it if you could leave a tip or share it with others who might benefit!
Looking for custom design work? Feel free to reach out—I’m available for freelance projects to help you create beautiful, functional websites.
Mycomponents: To download the templates, visit My Gumroad Page.
You can also read my recent posts