Views 185
How to add code in head/body/footer without plugin
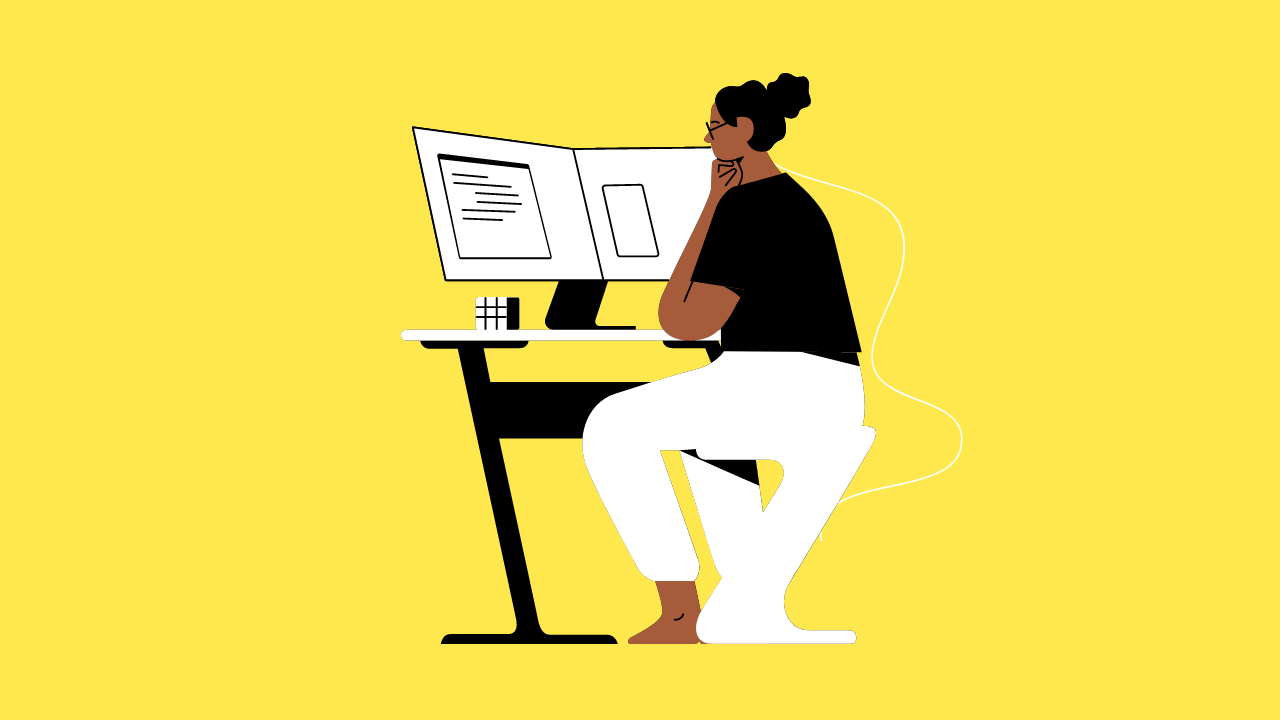
How to add code in head/body/footer without plugin – Adding custom code to the head, body, or footer of your WordPress site is often necessary for including tracking scripts, CSS, or JavaScript. While many users opt for plugins, you can do this without relying on one, resulting in cleaner code and fewer plugins to manage. Here’s a step-by-step guide to help you add custom code directly into your WordPress theme in a clean and safe way.
Table of Contents
1. Understanding Where to Place the Code
- Head section: Used for including meta tags, stylesheets, or other scripts that should load before the content of the page.
- Body section: Mainly used for inline JavaScript or HTML that affects the content directly after the page starts loading.
- Footer section: Ideal for adding JavaScript files and third-party tracking codes (e.g., Google Analytics) that should load after the content.
Before proceeding, ensure you’re working with a child theme to prevent losing your changes when the theme updates. If you’re unfamiliar with child themes, this guide explains how to create one.
2. Editing the functions.php
File
To add code to the head, body, or footer, you can use the wp_head()
, wp_body_open()
, and wp_footer()
hooks in the functions.php
file of your theme or child theme. These hooks allow you to insert custom code into specific sections of your site without directly editing theme template files.
Adding Code to the Head
To add custom code to the <head>
section of your website, use the wp_head
action hook. This hook is automatically triggered in the <head>
of your theme’s template, usually in header.php
.
phpCopy code// Add custom code to the <head> section
function my_custom_head_code() {
echo '<!-- Custom code or scripts for the head -->';
echo '<meta name="description" content="This is a custom description">';
}
add_action('wp_head', 'my_custom_head_code');
In this example, a meta description is added to the head section.
Adding Code to the Body
WordPress 5.2 introduced the wp_body_open()
hook, which can be used to inject code right after the opening <body>
tag. If your theme doesn’t already support this hook, add it to the beginning of header.php
like this:
phpCopy code<body <?php body_class(); ?>>
<?php wp_body_open(); ?>
Then, in your functions.php
file, you can add your custom code:
phpCopy code// Add custom code after the opening <body> tag
function my_custom_body_open_code() {
echo '<!-- Custom code for the body -->';
echo '<div class="custom-banner">Welcome to my site!</div>';
}
add_action('wp_body_open', 'my_custom_body_open_code');
This will display a custom banner right after the <body>
tag.
Adding Code to the Footer
To insert custom code or scripts before the closing </body>
tag, use the wp_footer()
hook. This is especially useful for scripts like Google Analytics or other third-party services.
phpCopy code// Add custom code to the footer
function my_custom_footer_code() {
echo '<!-- Custom footer code -->';
echo '<script type="text/javascript">console.log("Footer loaded");</script>';
}
add_action('wp_footer', 'my_custom_footer_code');
This code will add a custom JavaScript function that runs when the page is loaded.
3. Best Practices for Adding Code
- Avoid hard-coding scripts directly in template files: Always use hooks in
functions.php
for better maintainability. - Test in a staging environment: Before making changes on a live site, test the new code in a local or staging environment to avoid potential errors.
- Use a child theme: As mentioned earlier, using a child theme ensures that your changes won’t be lost when the parent theme is updated.
- Minify and defer scripts: When adding JavaScript, especially in the footer, consider minifying or deferring them for better page performance.
You can aslo read how to redirect a page in wordpress without plugin
4. Example Use Cases
Here are a few examples of what you can achieve by adding custom code to the head, body, or footer:
- Adding Google Analytics (in the footer):phpCopy code
function add_google_analytics() {
echo "<!-- Google Analytics -->
<script async src='https://www.googletagmanager.com/gtag/js?id=YOUR_TRACKING_ID'></script>
<script>
window.dataLayer = window.dataLayer || [];
function gtag(){dataLayer.push(arguments);}
gtag('js', new Date());
gtag('config', 'YOUR_TRACKING_ID');
</script>";
}
add_action('wp_footer', 'add_google_analytics');
- Including custom CSS (in the head):phpCopy code
function add_custom_css() { echo '<style> body { background-color: #f0f0f0; } </style>'; } add_action('wp_head', 'add_custom_css');
- Adding a banner after the opening body tag:phpCopy code
function add_body_banner() { echo '<div class="welcome-banner">Welcome to Our Website</div>'; } add_action('wp_body_open', 'add_body_banner');
5. Conclusion
By using the built-in WordPress hooks like wp_head()
, wp_body_open()
, and wp_footer()
, you can easily add custom code to your site without using a plugin. This method is cleaner, more efficient, and helps you maintain full control over your site’s performance and functionality. Remember to always back up your theme files before making any changes and test thoroughly to ensure everything works smoothly.
Feel free to experiment with these methods to achieve the desired result for your WordPress website!